Modify ImageViewViewController.h:
#import <UIKit/UIKit.h>
@interface ImageViewViewController : UIViewController {
UILabel *sliderValue;
}
@property (nonatomic, retain) IBOutlet UILabel *sliderValue;
- (IBAction)sliderChanged:(id)sender;
@end
Modify ImageViewViewController.m:
#import "ImageViewViewController.h"
@implementation ImageViewViewController
@synthesize sliderValue;
- (IBAction)sliderChanged:(id)sender {
UISlider *slider = (UISlider *)sender;
NSString *newText = [[NSString alloc] initWithFormat:@"%1.2f",
slider.value];
sliderValue.text = newText;
[newText release];
}
- (void)didReceiveMemoryWarning {
// Releases the view if it doesn't have a superview.
[super didReceiveMemoryWarning];
// Release any cached data, images, etc that aren't in use.
}
- (void)viewDidUnload {
// Release any retained subviews of the main view.
// e.g. self.myOutlet = nil;
}
- (void)dealloc {
[sliderValue release];
[super dealloc];
}
@end
Switch to Interface Builder to add the UI now, double click on ImageViewViewController.xib to start Interface Builder.
Add a Label and Slider (both from the Inputs & Values under Cocoa Touch of Library) on the View pane.
Now check the setting of the Slider: click on the Slider to select it, and click Tools -> Inspector from Interface Builder top menu.
Accept the default value of min = 0, max =1 and initial = 0.5...
Now double click on the Label to change the text to 0.5, to match with the initial value of the Slider.
Connect the Action Method of the Slider:
click on the Slider to select it, and click Tools -> Connections Inspector from Interface Builder top menu.
Drag the circle on the right of the event Value Changed, to over File's Owner in ImageViewViewController.xib, release and select sliderChanged.
Connect the Outlet to the Label:
Drag the File's Owner by right button on mouse in ImageViewViewController.xib to over the Label in View. Release and select sliderValue.
Save your work, and Build and Run in Xcode.
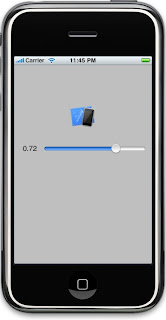
No comments:
Post a Comment